java时间戳转秒
发布时间:2023-06-15 16:53:00
发布人:zyh
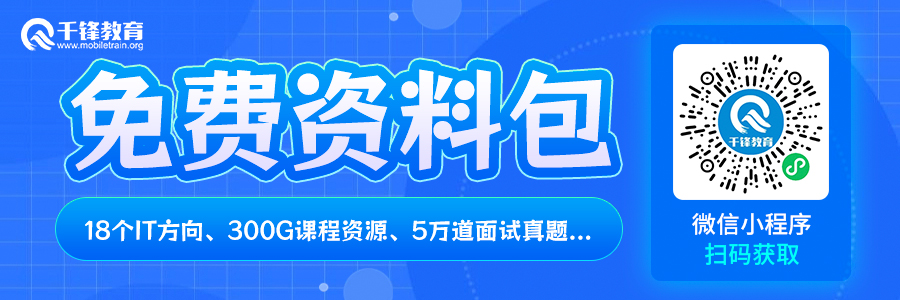
在Java中,可以通过将时间戳转换为秒数来实现。时间戳是指从特定的起始时间(通常是1970年1月1日 00:00:00 UTC)到特定时间点的经过的毫秒数。
下面是一种常见的方法,将时间戳转换为秒数的示例代码:
import java.time.Instant;
public class TimestampToSeconds {
public static void main(String[] args) {
long timestamp = 1623750000000L; // 示例时间戳
// 将时间戳转换为秒数
long seconds = timestamp / 1000;
System.out.println("Timestamp: " + timestamp);
System.out.println("Seconds: " + seconds);
}
}
在上述示例中,我们定义了一个时间戳`timestamp`,并将其除以1000来得到对应的秒数。注意要使用长整型数值后缀`L`来表示时间戳。然后,我们将转换后的秒数打印输出。
运行上述代码,将会输出时间戳和对应的秒数。
需要注意的是,上述示例假设时间戳是以毫秒为单位的。如果你的时间戳是以其他单位(如微秒)表示的,你需要相应地调整转换的方式。
另外,Java 8及更高版本还提供了`java.time.Instant`类,它可以用于处理时间戳和时间的转换。下面是使用`Instant`类将时间戳转换为秒数的示例代码:
import java.time.Instant;
public class TimestampToSeconds {
public static void main(String[] args) {
long timestamp = 1623750000000L; // 示例时间戳
Instant instant = Instant.ofEpochMilli(timestamp);
long seconds = instant.getEpochSecond();
System.out.println("Timestamp: " + timestamp);
System.out.println("Seconds: " + seconds);
}
}
在上述示例中,我们使用`Instant.ofEpochMilli()`方法将时间戳转换为`Instant`对象,然后使用`getEpochSecond()`方法获取对应的秒数。
无论是使用简单的除法运算还是使用`Instant`类,你都可以将时间戳转换为秒数,以满足你的需求。