21个面向Web开发人员的JavaScript技巧汇总
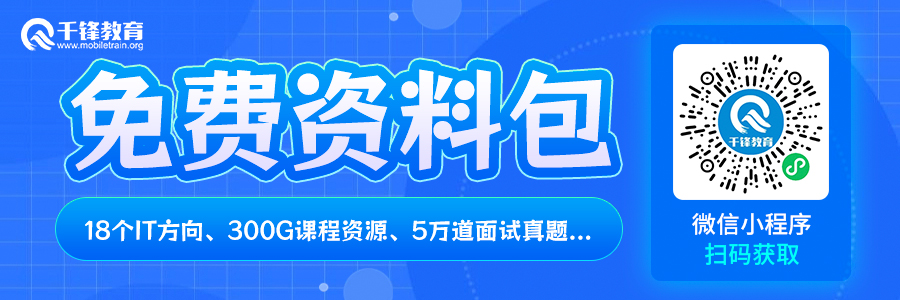
作为程序员,编写代码也需要大量的技巧。好的代码可以让人耳目一新、通俗易懂、舒适自然,同时又充满成就感。因此,整理了一些经常使用的JavaScript开发技巧,希望能让大家写出耳目一新、通俗易懂、舒适自然的代码。
**字符串技巧**
**1、比较时间**
```text
const time1 = "2022-03-02 09:00:00";
const time2 = "2022-03-02 09:00:01";
const overtime = time1 < time2;
// overtime => true
```
**2、格式化money**
```text
const ThousandNum = num => num.toString().replace(/\B(?=(\d{3})+(?!\d))/g, ",");
const money = ThousandNum(1000000);
// money => '1,000,000'
```
**3、生成随机ID**
```text
const RandomId = len => Math.random().toString(36).substr(3, len);
const id = RandomId(10);
// id => "xdeguewg1f"
```
**4、生成随机 HEX 颜色值**
```text
const RandomColor = () => "#" + Math.floor(Math.random() * 0xffffff).toString(16).padEnd(6, "0");
const color = RandomColor();
// color => "#2cbf89"
```
**5、Generate star ratings**
```text
const StartScore = rate => "★★★★★☆☆☆☆☆".slice(5 - rate, 10 - rate);
const start = StartScore(3);
// start => '★★★☆☆'
```
**6、网址查询参数**
```text
const params = new URLSearchParams(location.search.replace(/\?/ig, "")); // location.search = "?name=test&sex=man"
params.has("test"); // true
params.get("sex"); // "man"
```
**数字技能**
**7、Arrangement**
```text
用 Math.floor() 代替正数,用 Math.ceil() 代替负数
const num1 = ~~ 1.19;
const num2 = 2.29 | 0;
const num3 = 3.09 >> 0;
// num1 num2 num3 => 1 2 3
```
**8、零填充**
```text
const FillZero = (num, len) => num.toString().padStart(len, "0");
const num = FillZero(1234, 5);
// num => "01234"
```
**9、转数**
```text
仅对 null、“”、false、数字字符串有效
const num1 = +null;
const num2 = +"";
const num3 = +false;
const num4 = +"169";
// num1 num2 num3 num4 => 0 0 0 169
```
**10、时间戳**
```text
const timestamp = +new Date("2022-03-22");
// timestamp => 1647907200000
```
**11、精确小数**
```text
const RoundNum = (num, decimal) => Math.round(num * 10 ** decimal) / 10 ** decimal;
const num = RoundNum(1.2345, 2);
// num => 1.23
```
**12、平价**
```text
const OddEven = num => !!(num & 1) ? "odd" : "even";
const num = OddEven(2);
// num => "even"
```
**13、取最小值最大值**
```text
const arr = [0, 1, 2, 3];
const min = Math.min(...arr);
const max = Math.max(...arr);
// min max => 0 3
```
**14、生成范围随机数**
```text
const RandomNum = (min, max) => Math.floor(Math.random() * (max - min + 1)) + min;
const num = RandomNum(1, 10); // 5
布尔技能
```
**布尔技能**
**15、短路运算符**
```text
const a = d && 1; // Fake operation, judge from left to right, return a false value when encountering a false value, and no longer execute it later, otherwise return the last true value
const b = d || 1; // Take the true operation, judge from left to right, return the true value when encountering the true value, and do not execute it later, otherwise return the last false value
const c = !d; // Returns false if a single expression converts to true, otherwise returns true
```
**16、确定数据类型**
```text
可确定的类型:undefined、null、string、number、boolean、array、object、symbol、date、regexp、function、asyncfunction、arguments、set、map、weakset、weakmap
function DataType(tgt, type) {
const dataType = Object.prototype.toString.call(tgt).replace(/\[object (\w+)\]/, "$1").toLowerCase();
return type ? dataType === type : dataType;
}
DataType("test"); // "string"
DataType(20220314); // "number"
DataType(true); // "boolean"
DataType([], "array"); // true
DataType({}, "array"); // false
```
**17、检查数组是否为空**
```text
const arr = [];
const flag = Array.isArray(arr) && !arr.length;
// flag => true
18、满足条件时执行
const flagA = true; // Condition A
const flagB = false; // Condition B
(flagA || flagB) && Func(); // Execute when A or B is satisfied
(flagA || !flagB) && Func(); // Execute when A is satisfied or B is not satisfied
flagA && flagB && Func(); // Execute when both A and B are satisfied
flagA && !flagB && Func(); // Execute when A is satisfied and B is not satisfied
19、如果非假则执行
const flag = false; // undefined、null、""、0、false、NaN
!flag && Func();
```
**20、数组不为空时执行**
```text
const arr = [0, 1, 2];
arr.length && Func();
```
**21、对象不为空时执行**
```text
const obj = { a: 0, b: 1, c: 2 };
Object.keys(obj).length && Func();
```
**- End -**
更多关于“html5培训”的问题,欢迎咨询千锋教育在线名师。千锋已有十余年的培训经验,课程大纲更科学更专业,有针对零基础的就业班,有针对想提升技术的提升班,高品质课程助理你实现梦想。