python rpc调用方法总结
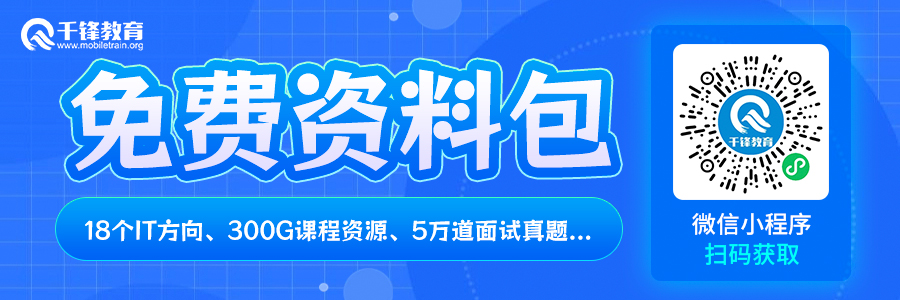
在 Python 中进行 RPC(远程过程调用)调用,你可以使用多种方法。以下是几种常见的 Python RPC 调用方法的总结:
1. XML-RPC:
XML-RPC 是一种使用 XML 格式进行远程过程调用的协议。Python 内置了 `xmlrpc.client` 模块,使得进行 XML-RPC 调用变得简单。你可以使用 `xmlrpc.client.ServerProxy` 类来创建 XML-RPC 代理对象,然后调用远程方法。
python
import xmlrpc.client
# 创建 XML-RPC 代理对象
proxy = xmlrpc.client.ServerProxy("http://example.com/api")
# 调用远程方法
result = proxy.method_name(arg1, arg2)
2. JSON-RPC:
JSON-RPC 是一种使用 JSON 格式进行远程过程调用的协议。Python 中有多个第三方库可以实现 JSON-RPC 调用,如 `jsonrpcclient`、`jsonrpclib` 等。你可以根据自己的需求选择合适的库进行 JSON-RPC 调用。
python
import jsonrpcclient
# 创建 JSON-RPC 代理对象
proxy = jsonrpcclient.Server("http://example.com/api")
# 调用远程方法
result = proxy.method_name(arg1, arg2)
3. gRPC:
gRPC 是一种高性能、跨语言的远程过程调用框架,它使用 Protocol Buffers(protobuf)进行序列化。Python 提供了 `grpc` 模块,可以用于创建和调用 gRPC 服务。你需要定义 gRPC 服务的接口和消息类型,并使用生成的客户端代码进行调用。
python
import grpc
import your_pb2
import your_pb2_grpc
# 创建 gRPC 通道
channel = grpc.insecure_channel("localhost:50051")
# 创建 gRPC 客户端
client = your_pb2_grpc.YourServiceStub(channel)
# 调用远程方法
response = client.MethodName(your_pb2.Request(arg1=arg1, arg2=arg2))
这些是 Python 中常见的几种 RPC 调用方法。具体使用哪种方法取决于你的需求和使用场景。每种方法都有其特定的优点和适用性,你可以根据项目需求选择合适的方法进行远程过程调用。